This is a list of various front-end plugins and visual components.
TreeView
https://jonmiles.github.io/bootstrap-treeview/
This plugin leverages the best of bootstrap framework and helps you display hierarchical tree structures using the JSON data set.
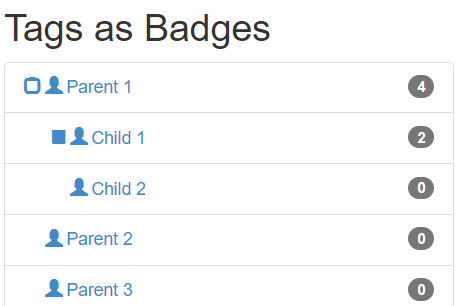
To read more, read this
Download from here
Bootstrap Material Select
Bootstrap Material Select is a form control that, after a click displays a collapsable list of multiple values which can be used in forms, menus or surveys.
For more info, read this
Bootstrap selectpicker
class
Add the selectpicker
class to your select elements to auto-initialize bootstrap-select.
<select class="selectpicker">
<option>Mustard</option>
<option>Ketchup</option>
<option>Barbecue</option>
</select>
Via JavaScript
// To style only selects with the my-select class
$('.my-select').selectpicker();
or
// To style all selects
$('select').selectpicker();
If calling bootstrap-select via JavaScript, you will need to wrap your code in a .ready()
block or place it at the bottom of the page (after the last instance of bootstrap-select).
Download from here