Shared projects are used to facilitate cross platform development. This allows you to reference an entire project as opposed to just a single assembly.
Shared project is a shred bucket of code. At compile time, any project that reference the shared project will have all of the files (including folder structure) and then they will be compiled. You wouldn’t see any separate DLL as you might have seen in PCL (Portable class libraries).
A shared project is not going to be compiled on its own. The code in the shared project is incorporated into assembly that reference it and compiled within that assembly.
Let’s create a shared project;
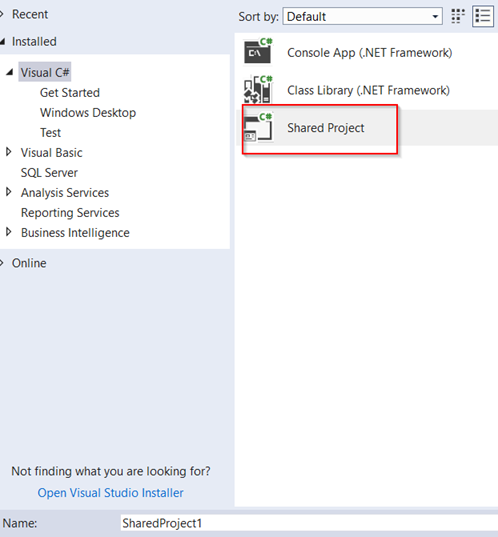
Create a class Math with a static method Add.
namespace SharedProject1
{
public class Math
{
public static int Add(int x, int y)
{
#if NETCOREAPP1_1
return (x + y) + 3;
#else
return (x + y) + 13;
#endif
}
}
}
Add SharedProject reference to your project. If your project is targeting .NET Core 1.1, the relevant piece of code in #if/#endif will run.
//.NET Core 1.1
SharedProject1.Math.Add(3, 4); //return 10
//.NET Core 1.0
SharedProject1.Math.Add(3, 4); //return 20
Here is some recommendation of using Shared Projects and Portable Class Libraries;
How the code is reused
- Shared Projects: Source Code (All source code is available to your reference project)
- PCL: Reference is available at Assembly level (for example MyLibrary.dll)
Compile time behavior
- Shared Projects: All source code is copied into each referenced project and compiled there
- PCL: Nothing new. Its compiled as usuall.
Visual Studio support
- Shared Projects: Full Support
- PCL: Each plateform is compiled separately. This can be accomplished thru IOC.
#IFDEF Support
- Shared Projects: Full Support
- PCL: Unsupported
.NET Framework Support
- Shared Projects: Full Support
- PCL: Limited
The core problem with shared project is difficulty of code testing because of conditional compilation directives. This in turn introduce errors that you wouldn’t know until you have actually compiled your application.
Resources
https://dev.to/rionmonster/sharing-is-caring-using-shared-projects-in-aspnet-e17

