For those that have never studied primitive vs reference types, let’s discuss the fundamental difference.
If a primitive type is assigned to a variable, we can think of that variable as containing the primitive value. Each primitive value is stored in a unique location in memory.
If we have two variables, x and y, and they both contain primitive data, then they are completely independent of each other:
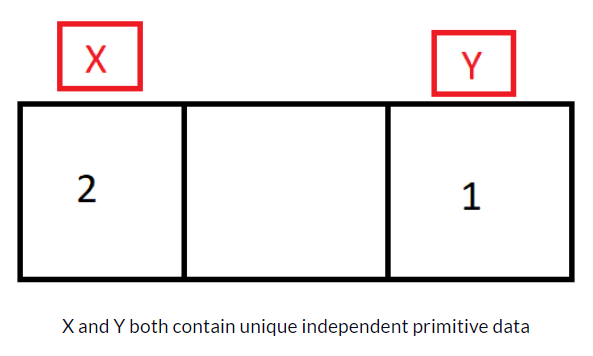
let x = 2;
let y = 1;
x = y;
y = 100;
console.log(x); // 1 (even though y changed to 100, x is still 1)
This isn’t the case with reference types. Reference types refer to a memory location where the object is stored.
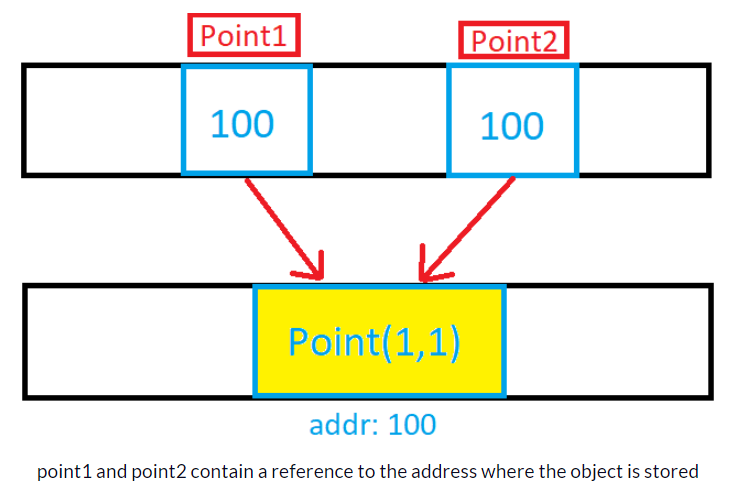
let point1 = { x: 1, y: 1 };
let point2 = point1;
point1.y = 100;
console.log(point2.y); // 100 (point1 and point2 refer to the same memory address where the point object is stored)
That was a quick overview of primary vs reference types.

