Let’s get our hands in some code. This web app will act as a monitoring and reporting service that will check the health status of a target server.
We are going to use Micorosft.AspNetCore.Diagnositcs.HealthChecks package, a built-in feature of the .NET Core framework. This package is meant to be used to allow a monitoring service to check the status of another running service.
Add app.UseHalthChecks middleware in Program.cs file;
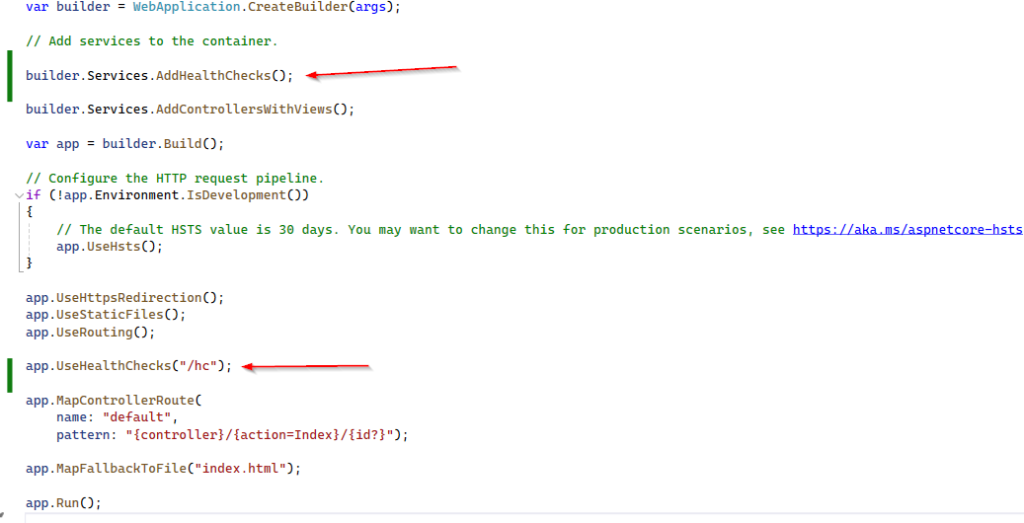
This middleware will create a server-side route for the health checks. Note that we have added this middleware before Endpoints so that this new route won’t be overriden by the general-purpose Controller route pattern.
Keep in mind, when we run application it uses two ports; one for server side and second one for angular side (in our case it’s 7031). For angular it creates a proxy and re-direct users there (in this case 44488).
If we run our application, we can see our system is healthy.
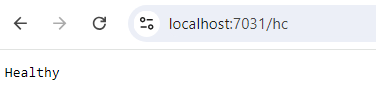
The system is healthy because we haven’t defined any checks yet.
Let’s add an Internet Control Message Protocol (ICMP) AKA Ping. PING request is a basic way to check the presence and therefore availability, of a server that we know we should be able to reach within a LAN / WAN connection.
In a nutshell, it works in following way; the machine that performs the PING sends one or more ICMP echo request packets to the target host and waits for a reply; if it receives it, it reports the round-trip time of the whole task; otherwise, it time outs and reports a “host not reachable” error.
The “host not reachable” error can be due to a number of possible reasons;
- The target host is not available
- The target host is available, but actively refuses TCP/IP connections of any kind
- The target host is available and accepts TCP/IP incoming conections, but it has been configured to explicitly refuse ICMP requests and/or not send ICMP echo replies back.
- The target host is available and properly configured to accept ICMP requests and send echo replies back, but the connection is very slow or hindered by unknown reasons (performance, heavy load etc), so the round-trip time takes too long, or even times out.
So what could be the possible outcomes of our implementation;
- Healthy: We can consider the host Healthy whenever the PING succeeded with no errors and timeouts.
- Degraded: We can consider the host Degraded whenever the PING succeeded, but the round-trip takes too long.
- Unhealthy: We can consider the host Unhealthy whenever the PING failed, that is, the check times out before any reply.
public class PingHealthCheck : IHealthCheck
{
private string Host = "www.does-not-exist.com";
private int Timeout = 300;
public async Task<HealthCheckResult> CheckHealthAsync(HealthCheckContext context, CancellationToken cancellationToken = default)
{
try
{
using (var ping = new Ping())
{
var reply = await ping.SendPingAsync(Host);
switch (reply.Status)
{
case IPStatus.Success:
return (reply.RoundtripTime > Timeout) ? HealthCheckResult.Degraded() : HealthCheckResult.Healthy();
default:
return HealthCheckResult.Unhealthy();
}
}
}
catch (Exception ex)
{
return HealthCheckResult.Unhealthy();
}
}
}
Add PingHealthCheck to the pipeline by modifying Program.cs file;
builder.Services.AddHealthChecks()
.AddCheck<PingHealthCheck>("ICMP");
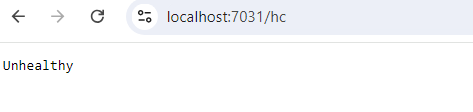
So we are getting a response. that’s great, it works. Three are 3 major flaws;
- Hardcoded values: The Host and the Timeout variables should be passed as parameters
- Uninformative response: Healthy and Unhealthy are not that great, we should create a better output message
- Untyped output: The current response is being sent in plain text, if we want to fetch it with Angular, a JSON content-type be better.
public class PingHealthCheck : IHealthCheck
{
private string Host { get; set; }
private int Timeout { get; set; }
public PingHealthCheck(string host, int timeout)
{
Host = host;
Timeout = timeout;
}
public async Task<HealthCheckResult> CheckHealthAsync(HealthCheckContext context, CancellationToken cancellationToken = default)
{
try
{
using (var ping = new Ping())
{
var reply = await ping.SendPingAsync(Host);
switch (reply.Status)
{
case IPStatus.Success:
var msg = $"ICMP to {Host} took {reply.RoundtripTime} ms.";
return (reply.RoundtripTime > Timeout) ? HealthCheckResult.Degraded(msg) : HealthCheckResult.Healthy(msg);
default:
var err = $"ICMP to {Host} failed: {reply.Status}";
return HealthCheckResult.Unhealthy(err);
}
}
}
catch (Exception ex)
{
var err = $"ICMP to {Host} failed: {ex.Message}";
return HealthCheckResult.Unhealthy(err);
}
}
}
Update middleware in Program.cs file so that we can call it programmatically;
builder.Services.AddHealthChecks()
.AddCheck("PING_01", new PingHealthCheck("www.msn.com", 100))
.AddCheck("PING_02", new PingHealthCheck("www.google.com", 100))
.AddCheck("PING_03", new PingHealthCheck("www.does-not-exist.com", 100));
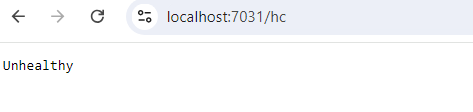
The reason is obvious, if one of them failed the whole stack will be failed. The last one in our example is failure. We can avoid this sum behavior by implementing the resolution of third flaw (as mentioned before): JSON-structured output message.
Add a new class in project root, CustomHealthCheckOptions;
public class CustomHealthCheckOptions : HealthCheckOptions
{
public CustomHealthCheckOptions() : base()
{
var jsonSerializerOptions = new JsonSerializerOptions()
{
WriteIndented = true
};
ResponseWriter = async (c, r) =>
{
c.Response.ContentType = MediaTypeNames.Application.Json;
c.Response.StatusCode = StatusCodes.Status200OK;
var result = JsonSerializer.Serialize(new
{
checks = r.Entries.Select(e => new
{
name = e.Key,
responseTime = e.Value.Duration.TotalMilliseconds,
status = e.Value.Status.ToString(),
description = e.Value.Description
}),
totalStatus = r.Status,
totalResponseTime = r.TotalDuration.TotalMilliseconds,
}, jsonSerializerOptions);
await c.Response.WriteAsync(result);
};
}
}
Modify Program.cs file;
app.UseHealthChecks("/hc", new CustomHealthCheckOptions());
Run the application and here is expected output;
{
"checks": [
{
"name": "PING_01",
"responseTime": 78.6889,
"status": "Healthy",
"description": "ICMP to www.msn.com took 12 ms."
},
{
"name": "PING_02",
"responseTime": 62.3402,
"status": "Healthy",
"description": "ICMP to www.google.com took 14 ms."
},
{
"name": "PING_03",
"responseTime": 144.9184,
"status": "Unhealthy",
"description": "ICMP to www.does-not-exist.com failed: An exception occurred during a Ping request."
}
],
"totalStatus": 0,
"totalResponseTime": 181.1726
}
Each and every check is properly documented, as well as the total outcome data, in a structured JSON object. This is just what we need to feed some Angular Components.
See in next article.

