The IEnumerable and IQueryable are used to hold a collection of data and also used to perform data manipulation operations such as filtering, Ordering, Grouping, etc.
The main difference is this;
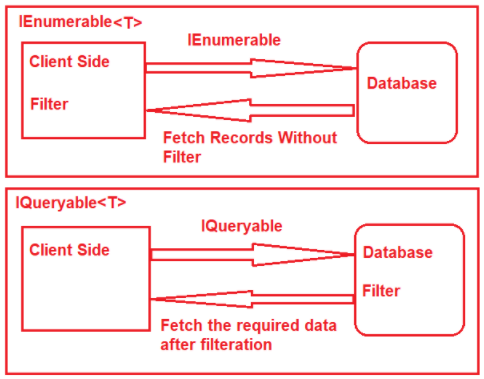
We can use SQL Profiler to confirm this behavior.
Source, Ingest, Prepare, Analyze and Consume
The IEnumerable and IQueryable are used to hold a collection of data and also used to perform data manipulation operations such as filtering, Ordering, Grouping, etc.
The main difference is this;
We can use SQL Profiler to confirm this behavior.
A short list of LINQ operators.
Using IN clause
This is similar to database IN keyword;
var myInClause = new string[] {"One", "Two", "Three"};
var results = from x in MyTable
where myInClause.Contains(x.SomeColumn)
select x;
// OR
var results = MyTable.Where(x => myInClause.Contains(x.SomeColumn));
Using ALL operator
Working with simple types
//does all numbers are greater than 10
int[] IntArray = { 11, 22, 33, 44, 55 };
var Result = IntArray.All(x => x > 10);
Console.WriteLine("Is All Numbers are greater than 10 : " + Result);
//does all names has characters greater than three
string[] stringArray = { "Khan", "Ali", "Adam", "Eve", "Joe" };
Result = stringArray.All(name => name.Length > 3);
Console.WriteLine("Is All Names are greater than 3 Characters : " + Result);
var letterResult = stringArray.All(name => name.StartsWith("A"));
Console.WriteLine("Is All Names start with letter A : " + letterResult);
//all numbers can be divided by three
int[] numbers = { 3, 6, 9, 12};
bool iSNumbersDivided = numbers.All(number => number % 3 == 0);
Console.WriteLine($"Numbers are divisible by three = {iSNumbersDivided}");
Working with complex types
//Check whether age of all animals in the zoo is greater than 1 year
bool response = animalData.All(x => x.AnimalAge > 1);
Console.WriteLine($"Is All Animals are greater than 1 years old : {response}");
//get all animas who are feed by milk
var zooSubSet = animalData.Where(x => x.Food.All(y => y.FoodType == "Milk"));
foreach(var item in zooSubSet)
{
Console.WriteLine($"Animal Name: {item.AnimalName}");
}
Resources
https://stackoverflow.com/questions/959752/where-in-clause-in-linq
https://coderedirect.com/questions/644629/linq-nested-list-contains
I am receiving this dataset from a remote server;
TagNumber TagCode CageNumber FoodType FoodValue
A-2021-1 WHITE A9:I10 Milk A11:I
A-2021-1 WHITE A9:I10 Corn A10:I
A-2021-1 RED A11:I13 Meat B1:B2
A-2021-1 RED A11:I13 Hay A14:I
A-2021-1 GREEN A8:J9 Milk B1:B2
A-2021-1 GREEN A8:J9 Milk A10:J
I need to create this object, a complex type;
public class Animal
{
public string TagNumber { get; set; }
public string TagCode { get; set; }
public string CageNumber { get; set; }
public List<AnimalFood> Food { get; set; }
}
public class AnimalFood
{
public string FoodType { get; set; }
public string FoodValue { get; set; }
}
There could be many ways to handle this. This is one of them;
Create a dictionary object using LINQ GroupBy and TagCode column from incoming dataset;
var allAnimals = animalData
.GroupBy(item => item.TagCode)
.ToDictionary(grp => grp.Key, grp => grp.ToList());
Create unique animals out of dictionary object;
var uniqueAnimals = allAnimals.Keys.Distinct().ToList();
Create the object (animal) and add them to zoo :);
var zoo = new List<Animal>();
foreach (string animal in uniqueAnimals)
{
var animalRow = allAnimals[animal];
var animalRowFirst = animalRow.FirstOrDefault();
var animalRecord = new Animal
{
TagNumber = animalRowFirst.TagNumber,
TagCode = animalRowFirst.TagCode,
CageNumber = animalRowFirst.CageNumber
};
//time for food
var animalFood = new List<AnimalFood>();
foreach(var item in animalRow)
{
item.Food.ForEach(x =>
{
animalFood.Add(new AnimalFood
{
FoodType = x.FoodType,
FoodValue = x.FoodValue
});
});
}
//this is our custom type
animalRecord.Food = animalFood;
zoo.Add(animalRecord);
}
Sample output;
It’s easier to apply different filters on this object;
var animalFilter = zoo.Where(x => x.TagCode.ToUpper() == "WHITE");
Resources
https://stackoverflow.com/questions/3186818/unique-list-of-items-using-linq
C# provides various ways to check for a null object.
Let’s create a class to test;
public class UserObject
{
public int Id { get; set; }
public string? Name { get; set; }
}
The most conventional way to check for null is by equating the object with null.
UserObject userObject = null;
//Conventional way to check for null
if (userObject == null)
{
userObject = new UserObject();
Console.WriteLine("userObject null - handled using conventional manner");
}
if (userObject != null)
{
Console.WriteLine("userObject not null - handled using conventional manner");
}
C#7 introduced a new way to write the above code in a more readable way by using the is
keyword.
//C#7 introduces a new way
userObject = null;
if (userObject is null)
{
userObject = new UserObject();
Console.WriteLine("userObject null - handled using c#7");
}
The same code can be written using null-coalescing operator.
//we can write the same code using null-coalescing operator
userObject = null;
userObject = userObject ?? new UserObject();
Console.WriteLine("userObject null - handled using null-coalescing operator");
C#9 introduced a new way to write the above code in a more readable way by using the is not
keyword.
//C#9 introduces a new way
if (userObject is not null)
{
userObject = new UserObject();
Console.WriteLine("userObject not null - handled using c#9");
}
Hope, this helps.
Design pattern provides a general reusable solution for the common problems that occur in software design. The pattern typically shows relationships and interactions between classes or objects.
These patterns are categorized into Structure, Creation and Behavior. This is a list of design pattern and their practical implementations.
Template Method Design Pattern
In simple words, a template method design pattern is a behavioral design pattern that defines the basic skeleton (steps) of an algorithm. In this pattern, we can change the behavior of individual steps in the derived class but can not change the sequence by which each method will be called
The real world examples are;
Builder Pattern
As the name suggests, this design pattern is used to build complex objects in a step by step approach. Using the same construction process we can make different representations of the object.
Suppose we have to build an object with a lot of different configurations based on a scenario. For example, we have to send an Email where we have optional properties like CC (carbon copy), BCC (blind carbon copy), attachments etc.
The real world examples are;